Day 14 - Tech Stacks for Frontend, Part 2
Frontend frameworks demystified. A deep-dive into React, Angular, and Vue.js for Product Managers
In the first part of the series on Tech Stacks, we talked about how they are like pieces of Lego and why general knowledge about them is helpful for Product Managers.
In today's post, we'll cover popular front-end frameworks and some key features about them.
Before we jump in
If you have noticed, not only do I want to describe the concept we are talking about (the "What"), but I would like to give an idea of the "why" we need them in the first place too.
This way, you can remember knowledge for a longer time as well and when faced with a new instance of the same concept, in this case, a new web framework, you will grasp it much easier and independently.
Recap on what is a software development framework
Software development frameworks provide a set of tools and standards for developing software applications.
These frameworks can help simplify the development process by providing a common structure for the code, as well as pre-built modules and components that can be used to implement common functionality. This can save time and effort for developers, and can also help ensure that the resulting software is more consistent and maintainable.
Some examples of software development frameworks include Ruby on Rails for web applications, Angular for front-end web development, and TensorFlow for machine learning.
Frontend Frameworks
Keeping the quest for the "why" in mind, let's explore briefly why we need a front-end framework in the first place. Why not simple HTML, CSS, and Javascript not enough to build modern web applications?
The What
Frontend frameworks are used to facilitate the development of user interfaces for web applications. These frameworks provide a set of pre-written code and tools that can be used to build user interfaces that are consistent, responsive, and easy to use.
Pre-framework era
It's a very rough division, but let's go back to the time when using frameworks was not common among developers, either they didn't exist or they were too young and companies haven't had the chance to migrate or create a new application based on them.
Before the existence of front-end frameworks, developers had to build user interfaces from scratch, which required a lot of time and effort. This often resulted in user interfaces that were difficult to maintain and scale, and that lacked consistent design and functionality. In short, a lot of headaches and a very long time to market.
It is also worth looking into the design of popular websites back in, let's say 2005. I'll let you explore them by yourself here:
Web Design MuseumWeb Design Museum
Or this gold mine of Best websites according to Time in 2005.
With the advent of frontend frameworks, developers can now build user interfaces more quickly and easily by using pre-written code and components provided by the framework. This can help to improve the consistency, responsiveness, and overall quality of the user interface.
It can also make the development process more efficient, as developers can reuse and extend the components provided by the framework instead of having to write everything from scratch.
As a result, frontend frameworks have become an essential part of modern web development.
If you remember from Day 9, we talked about the website that surveys different Javascript frameworks and technologies, called State of JS.
Now let's look into the popular frameworks for Frontend and explore them. We have to rely on 2021 data because the survey for 2022 is ongoing.
💡 If you are a software developer, head to the survey to provide data 🙌🏻
Also, note the rise of Svelte in the charts. I'm very curious about the results for 2022 🧐. You can get a hint from the trends on StackOverflow too:
React
React is a JavaScript library for building user interfaces. It was created by Meta (Facebook back then) in May 2013 and is used by many companies and developers to build web applications.
React's core concept is the use of components to build user interfaces. In React, a component is a piece of code that represents a part of the user interface, such as a button or a form. Components can be reused and composed to build complex user interfaces, which makes the development process more efficient and modular.
One of the main reasons to use React is its efficiency. React uses a virtual DOM (Document Object Model) to update the user interface, which makes the process of rendering components to the screen faster and more efficient. This can help to improve the performance of web applications, especially those with large and complex user interfaces.
Another advantage of React is its flexibility. React components can be used in different contexts, such as in a web browser, on a mobile device, or on a server. This allows developers to build applications that can run on multiple platforms, and to reuse components across different projects.
Some potential drawbacks of using React include its steep learning curve and the need to write code in JavaScript or Typescript.
React also has a large ecosystem of third-party libraries and tools, which can make it difficult to choose the right ones for a given project. Overall, however, React is a popular and powerful tool for building user interfaces and is widely used by developers.
Components in React are written in .jsx
or .tsx
(if using Typescript) and it looks like this:
function Welcome(props) {
return <h1>Hello, {props.name}</h1>;
}
function App() {
return (
<div>
<Welcome name="Sara" />
<Welcome name="Cahal" />
<Welcome name="Edite" />
</div>
);
}
Angular
Angular is a JavaScript framework for building web applications and apps in JavaScript, HTML, and TypeScript. It was created by Google, kinda a competitor for React. Note that what we refer to as Angular nowadays is a successor to AngularJS (or more specifically AngularJS Version 2.0 and onward which was released on October 2014 is renamed to Angular, due to its huge overhaul).
The core concepts of Angular, just like React, include components, directives, and services. Components are the building blocks of Angular applications and are the most basic UI elements. Directives are markers on a DOM element that tells Angular to attach a specific behavior to that DOM element. Services are reusable pieces of code that can be injected into different components to provide specific functionality.
One reason to use Angular is that it allows for the creation of reusable components, which can make development faster and more efficient. Additionally, Angular offers strong support for building complex, large-scale applications, and has a large and active community.
Angular uses ng-*
HTML properties to connect code with HTLM elements. An example Angular code will look like:
<!DOCTYPE html>
<html>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js"></script>
<body>
<div ng-app="" ng-init="firstName='John'">
<p>The name is <span ng-bind="firstName"></span></p>
</div>
</body>
</html>
Vue.js
Vue.js is a JavaScript framework for building web applications and user interfaces. It was first created in February 2014 by Evan You, a former employee of Google who used Angular in several projects.
The core concepts of Vue.js, like Angular and React, include components, reactive data binding, and the virtual DOM.
Reactive data binding means that when data in the application changes, the user interface updates automatically. The virtual DOM is an in-memory representation of the actual DOM, which allows Vue.js to update the UI efficiently by only changing the parts of the DOM that have actually changed.
One reason to use Vue.js is that it is easy to learn and use, especially for developers who are already familiar with JavaScript. Vue.js also has a small size and good performance, making it well-suited for building fast, lightweight applications. Additionally, Vue.js has a strong focus on developer experience, with helpful error messages and a focus on making the code readable and maintainable.
Some potential drawbacks of using Vue.js include a relatively small community compared to other JavaScript frameworks, which can make it harder to find support and resources. Additionally, Vue.js may not be as well-suited for building large-scale, complex applications as other frameworks like Angular.
In comparison to Angular, Vue.js is generally considered to be easier to learn and use, with a shallower learning curve. Vue.js also has a smaller size and better performance than Angular. However, Angular has a larger and more active community and may be better suited for building larger, more complex applications.
In comparison to React, Vue.js and React have many similar features and capabilities. Both use components and a virtual DOM, and both have strong performance and a focus on developer experience. However, Vue.js has a more straightforward and intuitive API, which may make it easier to learn and use for some developers. React, on the other hand, has a larger community and a more robust ecosystem of third-party libraries and tools.
Comparison and Summary
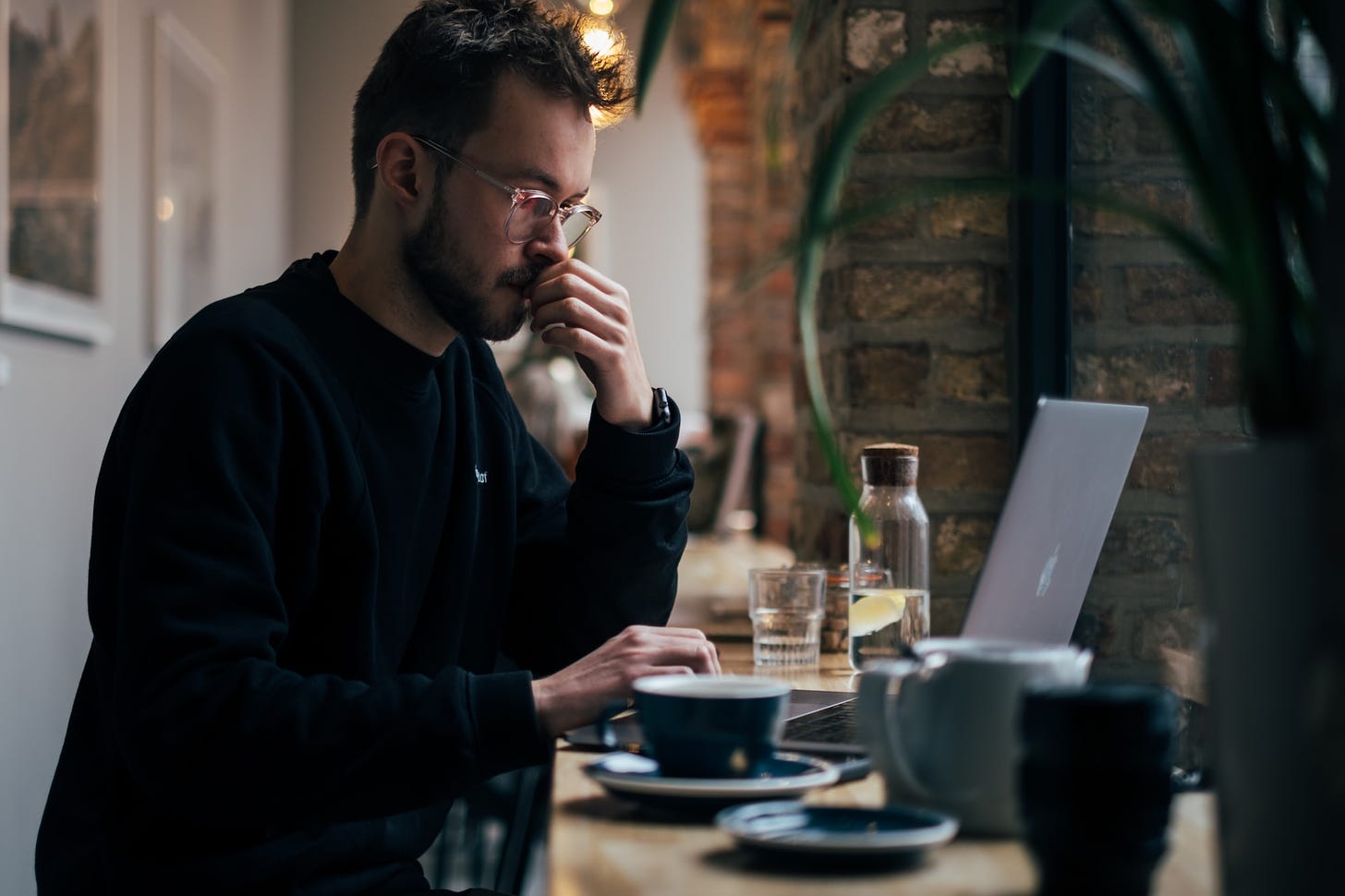
In the end, React, Angular, and Vue.js are all popular JavaScript frameworks for building web applications and user interfaces. All three frameworks have their own strengths and weaknesses, and the best choice for a particular project will depend on the specific requirements and use cases.
React is a good choice for building complex, large-scale applications, and has a large and active community. It is also well-suited for building applications that will be used on different platforms, such as web, mobile, and desktop.
Angular is a good choice for building large, complex applications, and has a large and active community. It offers a powerful set of tools and features and has strong support for building reusable components.
Vue.js is a good choice for building lightweight, fast applications, and has a focus on ease of use and developer experience. It is also a good choice for developers who are new to JavaScript frameworks, as it has a relatively shallow learning curve.
Overall, the best choice of the framework will depend on the specific requirements and use cases of the project. A developer should consider factors such as the size and complexity of the project, the desired performance and scalability, and the availability of resources and support from the community.
What do you think about Frontend frameworks? Which one are you using in your company?
Let me know in the comments below or reply to the email.